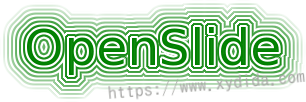
OpenSlide
OpenSlide is a C library that provides simple interfaces to process Whole-Slide-Images (also known as virtual slides). WSIs are very high resolution images used in digital pathology. These images usually have several gigabytes and can not be easily read with standard tools or libraries because the uncompressed images often occupy tens of gigabytes which consume huge of RAM. WSI is scanned with multi-resolution, while OpenSlide can support read a small amount of image data at the resolution closest to a specifical magnification level.
OpenSlide supports many popular WSI format:
- Aperio (.svs, .tif)
- Hamamatsu (.ndpi, .vms, .vmu)
- Leica (.scn)
- MIRAX (.mrxs)
- Philips (.tiff)
- Sakura (.svslide)
- Trestle (.tif)
- Ventana (.bif, .tif)
- Generic tiled TIFF (.tif)
Although it is written by C, Python and Java bindings are also provided, and some other bindings are contributed in the Github such as Ruby, Rust.
In this post, I’ll introduce how ot install OpenSlide
and OpenSlide-Python
binding in Windows
and Ubuntu
, and give some examples.
Windows install
OpenSlide
and OpenSlide-Python
are different,that must be installed seperately. OpenSlide
is the core library, while the other is the implementation of the interface.
OpenSlide Python document: https://openslide.org/api/python/
Download OpenSlide: https://openslide.org/download/
First, download OpenSlide windows binaries and extract them to a folder.
Sencond, install OpenSlide Python
package. It is not recommended to install OpenSlide Python
with conda
here, I have tried several times with conda
, but got the PackageNotFoundError:
To work around, use pip
to install:
1 | pip install openslide-python |
In my computer, openslide-python 1.2.0
is installed.
Then, according to the document, in Python
, import in a with os.add_dll_directory()
statement:
1 | # The path can also be read from a config file, etc. |
Problems
However, when importing the openslide’s python binding, I got the WinError 127
, here is my error message:
1 | --------------------------------------------------------------------------- |
And it alerted “Entry point not found”:
I was stucked in this problem and has tried just every solution I found with no success.
Until I found a solution in Stackoverflow, It may seems that I was trapped in “DLL Hell”.
There is a Windows DLL file that is taking precedence over the DLL file python needs in this source code. You need to prepend the PATH variable in your Python binding module before calling the C openslide library with the full path of the bin folder where the C openslide library DLL files reside. This will override the Windows “zlib1.dll” file by using the OpenSlide “zlib1.dll” file. -author: MacGyver
So, I tried to prepend openslide bin
path in the PATH
environment. It did work! The whole import code:
1 | # The path can also be read from a config file, etc. |
Ubuntu install
If you have root
permission, you can install openslide
directly by apt
:
1 |
|
Just follow the openslide document, https://openslide.org/download/#distribution-packages
But, if you are in a restricted environment where you don’t have the permission to install with apt
, conda
is an altenative method.
Here, I will use miniconda
which is a minimal installer for conda (https://docs.conda.io/en/latest/miniconda.html).
1 | wget https://repo.anaconda.com/miniconda/Miniconda3-latest-Linux-x86_64.sh |
The commands above will download the latest miniconda and setup a $conda
local variable for the convinent. Then create an environment called ‘openslide’. If you want to add other packages or specific python version, visit https://docs.conda.io/projects/conda/en/latest/user-guide/tasks/manage-environments.html#viewing-a-list-of-your-environments
Until now, it works smoothly. But reached out an error when activated the environment.
Follow the instruction, I closed and reconnected to the shell, the base
environment was activated successfully. It doesn’t matter because I can install openslide with conda.
Run one of the following, https://anaconda.org/conda-forge/openslide:
1 | conda install -c conda-forge openslide |
Then, let’s install openslide-python
:
1 | pip install openslide-python |
Check it:
And import openslide:
WSI operation
Now, I can read the Whole-Slide-Images with OpenSlide.
read slide and close
1 | slide = openslide.OpenSlide(r'F17-011490.ndpi') |
If there is no error and get the same result, congratulations that it works!
The slide object has some useful functions to use, such as level_count
return total number of level, level_dimensions
return the dimension for thar target level of the slide.
In my task, I need to read a small part in the WSI at the target level, OpenSlide provides read_region(location, level, size)
that can simplify my work.
1 | slide = openslide.OpenSlide(r'F17-011490.ndpi') |
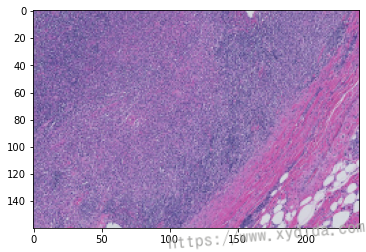
For the full usage, please visit https://openslide.org/api/python/#basic-usage
Happy coding.